Custom functions in Workflow Builder: Scheduling meetings across time zones
Leveraging Workflow Builder to simplify tasks
Difficulty
Beginner
Time
25 minutes
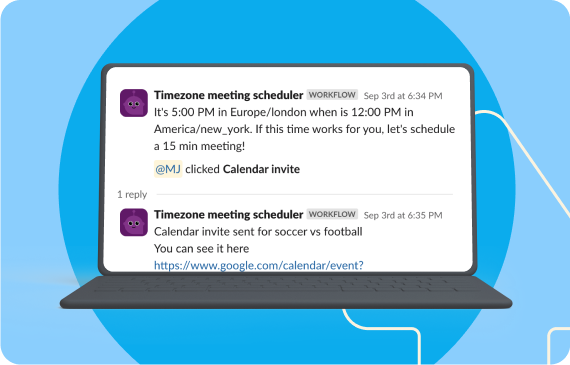
Requirements
- VsCode
- Slack CLI
- OR Github account (For a cloud development environment)
- A testing workspace (get an Enterprise Grid sandbox for free by joining developer program)
- A Google Calendar account
Quick Jump
- Step 1: Environment setup
- Step 2: Manifest
- Step 3: Create your custom function
- Step 4: Run the custom function
- Step 5: Choose your automation trigger
- Step 6: Collect meeting information
- Step 7: Add your custom function to Workflow Builder
- Step 8: Send a message to confirm the time conversion
- Step 9: Use the Google Calendar connector
- Step 10: Confirm with a message in thread
- Step 11: Test your function
- Step 12: Deployment
- Step 13: Troubleshooting
Build a timezone-aware meeting scheduler app
Coordinating meetings across different time zones can be a logistical headache. When you’re trying to schedule a meeting with a colleague or customer in another part of the world, it can be really difficult to find a time that works best for both of you. It requires switching between apps and doing mental calculations to convert time zones, which is tedious and makes it easy to make mistakes.
You can streamline this process directly within Slack. In this tutorial, you’ll build a workflow that automatically converts meeting times from one timezone to another, making it easier to schedule meetings with your global team members.
Using Slack’s Workflow Builder, you’ll create an automation that takes a proposed meeting time in your timezone, converts it to the timezone of the person you’re scheduling with, and then schedules the meeting. This workflow saves you time, reduces the chance of error, and allows you to focus on what matters most: collaborating with your team, no matter where they are in the world.
Timezone scheduler workflow at a glance
Your timezone scheduler workflow will consist of the following steps:
-
- Built-in function: Collect info in a form
- Custom function: Timezone aware meeting schedule
- Built-in function: Send a message with the time conversion and a button to create the calendar invite
- Calendar connector: Use the Google calendar connector to create the meeting
- Built-in function: Reply a message in thread with the confirmation of the meeting creation
Before we start, let’s review the tools we need. We’re going to combine the power and simplicity of Workflow Builder with the customizable opportunities of modular apps.
Workflow Builder is a no-code tool in Slack that allows users to automate tasks and processes by creating workflows. Workflows are collections of automated steps that are triggered by events, like when a message is posted in a channel. You can access Workflow Builder via the Automations menu on the left sidebar in Slack.
It’s easy for anyone in your organization to create Workflows that automate all kinds of tasks without writing code. If you have a problem that requires a custom solution, you can write a custom function in TypeScript that can then be used as a step in any workflow. And you can manage and deploy that function quickly and easily using the Slack command line tool.
Part I: Create your custom function (Steps 1-4)
Part II: Build your workflow step by step (Steps 5-12)
Step 1
Environment setup
You can follow this tutorial on your local machine or use Github codespaces cloud environment.
Option A. Local development
Install the Slack CLI following these instructions and clone the repo with the following command:
> slack create -t https://github.com/MJHR89/timezone-meeting-scheduler-template.git my-app
> cd my-app
Option B. Cloud development with Codespaces
Navigate to http://sfdc.co/Qkbve.
- Click the green code button.
- Click Codespaces.
- Click Create codespace on main.
This will set up your Codespace environment and install the Slack CLI and the Deno Slack SDK.
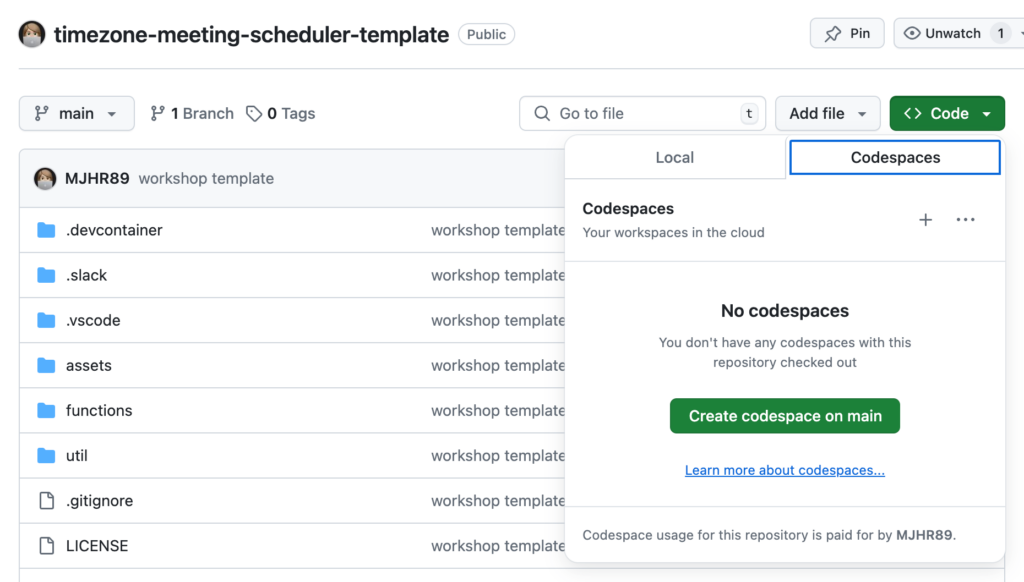
Test the Slack CLI installation
- In your Codespace terminal (or local terminal), run the command: slack version.
- If the installation was successful, you should see a version number displayed.
2. Connect Slack CLI with your Slack Workspace:
In the Codespace (or local) terminal, run: slack login. Follow the prompts to authenticate.
- Copy the command that looks like /slackauthticket THISISYOURTOKEN123456789 (including the /).
- Paste this command into any Slack channel. This will trigger a modal asking for permission.
- After accepting, copy the challenge code provided.
- Paste the challenge code back into your (Codespace) terminal.
You’re now authenticated! If you encounter any issues, refer to our documentation on authentication.
Step 2
Manifest
The manifest is where your app’s configurations are stored. Learn more in the Slack app manifest API documentation.
1. Add function and import statement
Add the function import and update the function list in your manifest file.
import { TimeZoneSchedulerFunction } from "./functions/timezone_scheduler_function.ts";
functions: [TimeZoneSchedulerFunction],
2. Add timeapi.io as outgoing domain
Deno runtime requires explicit permission to access external resources as a security measure. Add timeapi.io to the outgoingDomains array to allow your app to call the time conversion API.
outgoingDomains: ["timeapi.io"],
3. Name your app
Give your app a unique name so you can easily identify it.
name: "MJ-timezone-scheduler",
Step 3
Create your custom function
A custom function in Slack requires two key components:
- The function definition
- The function logic (handler)
1: Define the function
Navigate to functions/timezone_scheduler_function.ts and define the input and output parameters for your function. Ensure all parameters are included (there are some missing in the code) and properly defined as required. The parameters should follow this structure:
Input parameters: (you should have 5 in total)
- meeting_time: string (required)
- user_timezone: string (required)
- from_timezone: string (required)
- target_timezone: string (required)
- duration_minutes: number (required)
Output parameters: (you should have 4 in total)
- readable_time_origin: string (required)
- readable_time_participant: string (required)
- calendar_meeting_time: timestamp (slack type) (required)
- calendar_end_time: timestamp (slack type) (required)
2. Implement the function logic (function handler)
The SlackFunction()
method requires two arguments: the function’s definition and its logic.
- Pass the function definition as the first argument:
SlackFunction(TimeZoneSchedulerFunction, ...)
. - Map the inputs within your function.
- You can declare them together, for example:
const {
meeting_time,
from_timezone,
target_timezone,
duration_minutes,
user_timezone,
} = inputs;
- Or, use them directly as needed in your code, like:
inputs.meeting_time
.
Follow the instructions in the code to know where to use/insert each one.
- Import necessary helper functions for API calls and date formatting (check out the files in /util to know what they do).
import { convertTimeZone } from "../util/convert_timezone.ts";
import { formatDateTimeForAPI } from "../util/api_date_formatter.ts";
- Finally, ensure that each output parameter is correctly set to be used in subsequent workflow steps.
outputs: {
readable_time_origin: readableTimeOrigin,
readable_time_participant: readableTimeParticipant,
calendar_meeting_time: calendarMeetingTime,
calendar_end_time: calendarEndTime,
},
Step 4
Run the custom function
- In your Codespace terminal, run the command:
slack run
. - Select the Slack Workspace you authenticated with earlier (Step 1.2).
- If successful, you’ll see a message:
Connected awaiting events
.
Step 5
Choose your automation trigger
We’ll start by opening Workflow Builder. For this example, we’ll use a Link Trigger, which allows users to start the workflow on demand by clicking a link.
Starts from a link in Slack
Step 6
Collect meeting information
First, add a form to gather all the necessary details about the meeting. In Workflow Builder select the Form step from the sidebar menu.
Form Fields:
- From which timezone (e.g. Europe/London) → short answer
- What time and date → date/time picker
- How long (in minutes) → number
- To which timezone (e.g America/New_York) → short answer
- What’s your meeting about? → short answer
- Email of your contact → short answer
- (timezone where the workflow is being ran, e.g America/Los_Angeles) → short answer
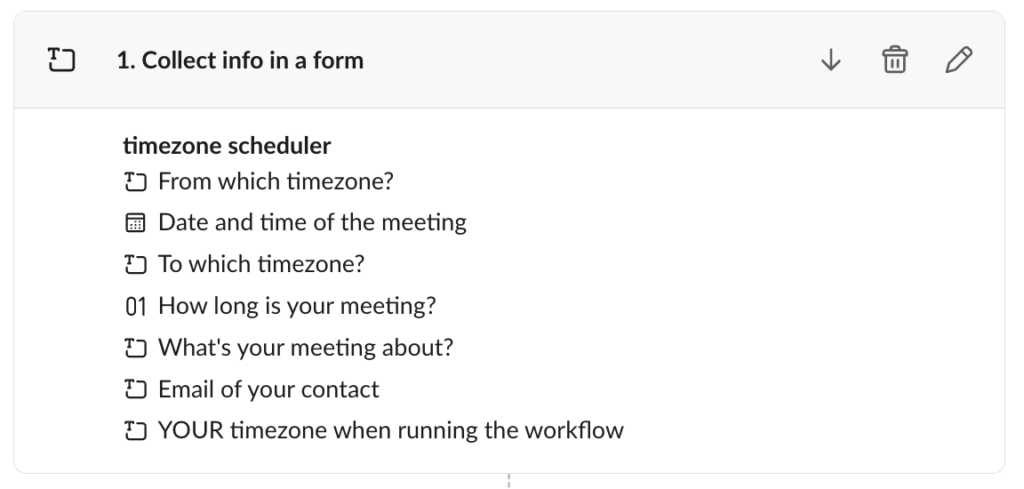
Step 7
Add your custom function to Workflow Builder
With your custom function now available in Workflow Builder (after running slack run
), add it as the second step:
- On the Steps sidebar, find your app or click on 🔧 Custom.
- Select Time Zone-Aware Meeting Scheduler as the step.
We need to connect the info we collected on the previous step to pass it as input of our function. For that we’re going to use some variables.
- Link the inputs from the form to your function. Click on the {} on the right side of the inputs, and choose the following (remember that the naming depends on what you’ve added in the form on step 1):
- Meeting time:
{}Answer to: Date and time of the meeting?
Then click on the caret v and choose the local compact format (that’s the format we expect in the code of our custom function) - User Timezone:
{}Answer to: Your timezone when running the workflow
- From timezone:
{}Answer to: From which timezone
- Target timezone:
{}Answer to: To which timezone
- Duration in minutes:
{}Answer to: How long
- Meeting time:
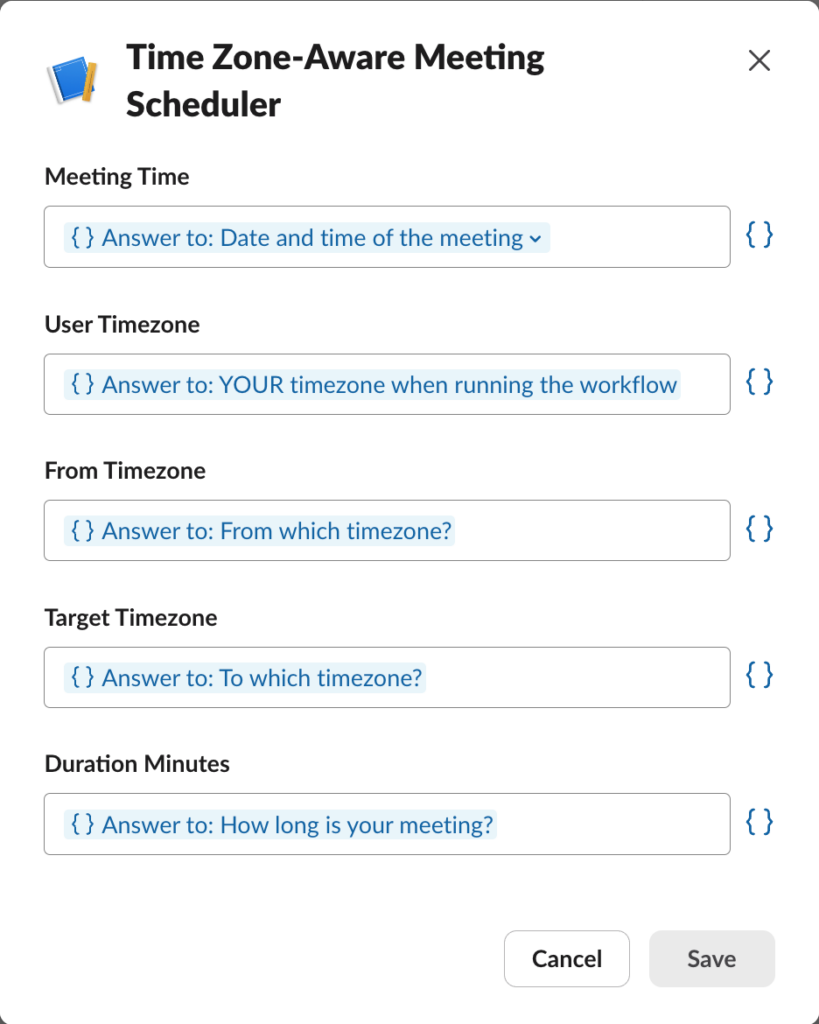
Step 8
Send a message to confirm the time conversion
Now, inform the user of the time conversion results. On the Steps sidebar, search for Messages > Send a message to a person.
You can craft your own message using all the variables available from previous steps. Here’s an example:
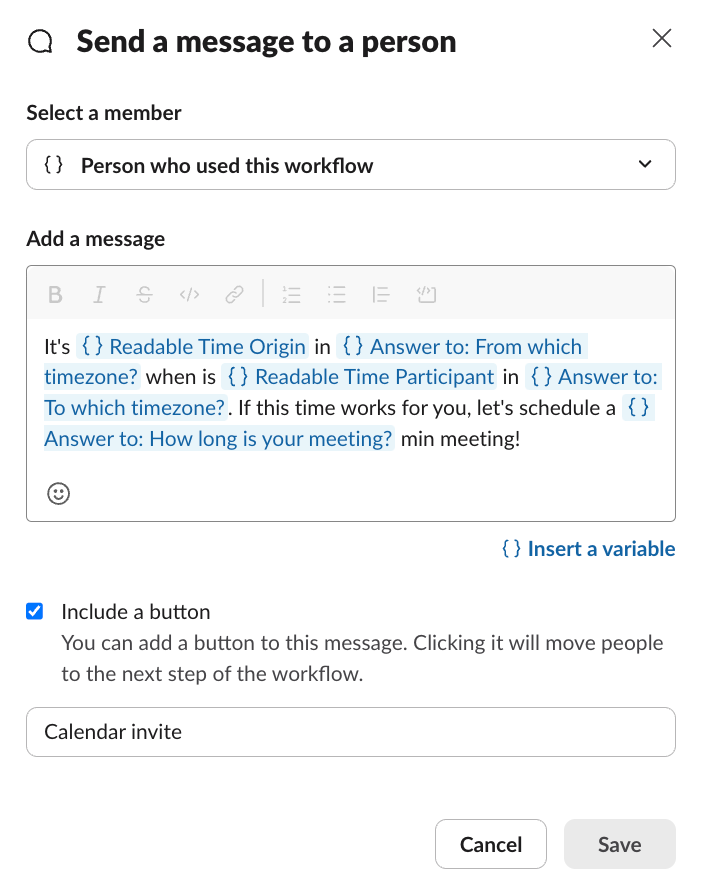
Enable the Include a button option and customize the button label.
The workflow will pause here and continue once the user clicks the button.
Step 9
Use the Google Calendar connector
Let’s add a connector for Google Calendar. On the Steps sidebar, search for Google Calendar > Create a calendar event. Fill in the event details using the variables from previous steps.
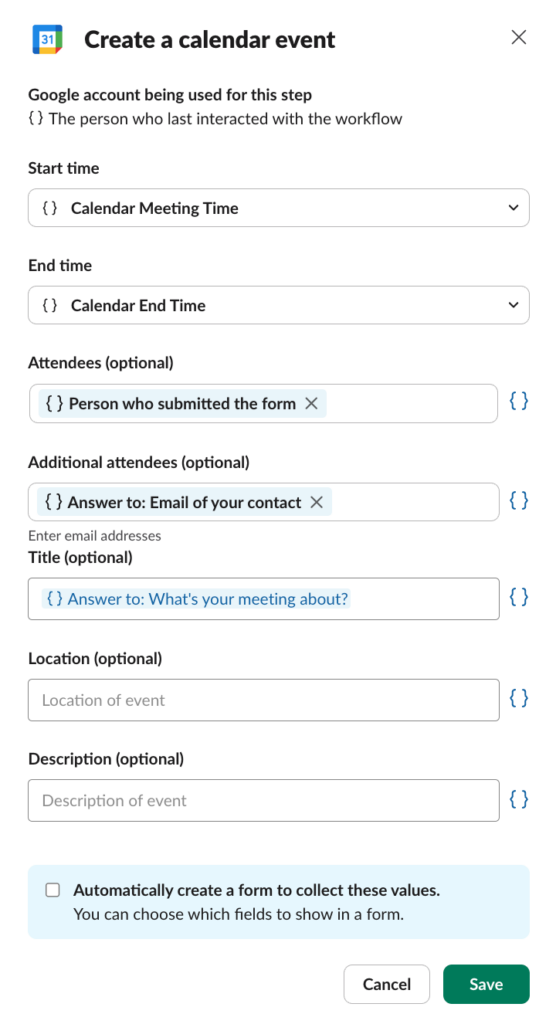
Step 10
Confirm with a message in thread
To finish, let the user know the event has been successfully scheduled.
On the Steps sidebar, search for Messages > Reply to a message in thread.
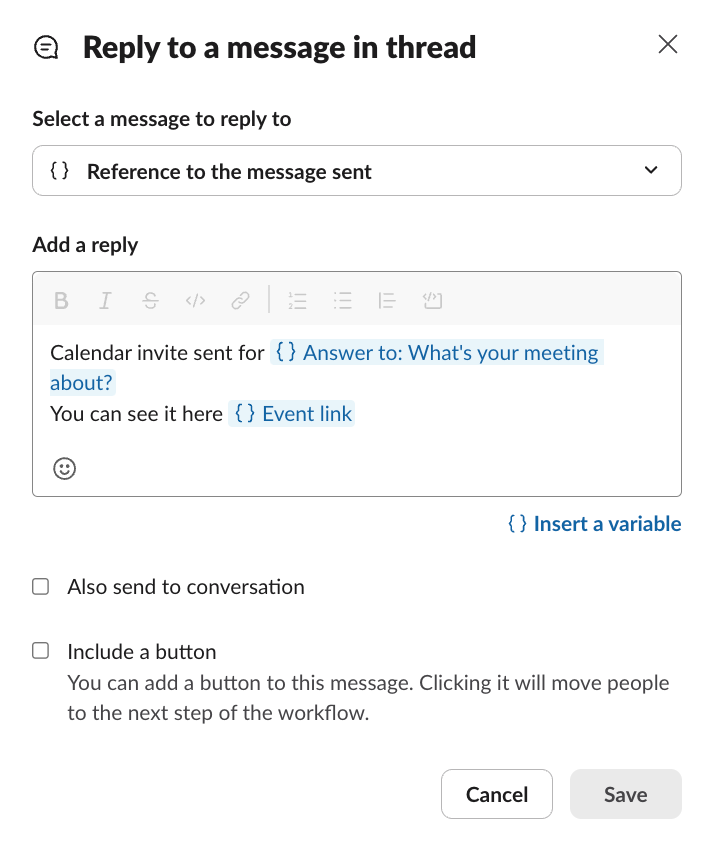
Step 11
Test your function
Your workflow is now complete! Click the Publish button in Workflow Builder, name your workflow, and copy the generated link trigger. Share the link in your test Slack channel, add it as a bookmark or to your channel canvas.
Run the workflow by clicking the link. You can find the full list of possible timezones under the util folder > timezones.txt.
When is your next meeting? 🌎🗓️
Step 12
Deployment
In the process of developing your function, you ran it in the GitHub Codespaces environment or your local machine, but as soon as you shut that environment down or turn off your computer, the function will stop running. Deploying your function to infrastructure managed by Slack ensures that the function is always available. Please note that there may be a cost associated with deploying functions to Slack, similar to using any serverless hosting environment.
To deploy your function to production simply run: slack deploy
.
Choose the appropriate workspace for deployment. Remember, the local version (slack run
) and the production version (slack deploy) are separate. Update the Workflow Builder to use the production function and reconnect input variables.
Step 13
Troubleshooting
Developers: If you encounter any issue with your custom function and need to debug, you can find the complete code in this repository: https://github.com/MJHR89/timezone-meeting-scheduler-final
Admins: If you’re new to coding but want to test the Part II of this tutorial, you can use codespaces to deploy this code (see Step 1, and Step 12 only) and start building your automation from Step 5.