Checking the weather using an automation in Slack: Automating workflows with the Slack platform
Reducing context switching by automating in Slack
Difficulty
Beginner
Time
20 minutes
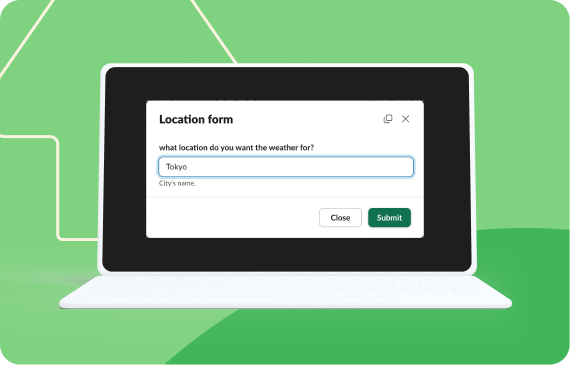
Requirements
- VsCode
- Slack CLI
- OpenWeather API account
- A testing workspace (get an Enterprise Grid sandbox for free by joining developer program)
Quick Jump
- Step 1: Mise en place (Everything in its place)
- Step 2: Creating your Slack project
- Step 3: Creating an account with OpenWeather
- Step 4: Creating a workflow with Workflow Builder
- Step 5: Adding a form Workflow step
- Step 6: Calling OpenWeather API
- Step 7: Configuring your manifest.ts file
- Step 8: Running your Slack app, and connecting it to the workflow
- Step 9: Send a message with your weather result
- Step 10: Extra credit (more learning!)
- Step 11: Deploy your weather app
Checking the weather directly in Slack
In today’s modern world, work happens wherever you can get a good connection to the internet, and that could be your home, the office or at your favorite coffee shop. The thing is, who wants to go outside when it is pouring rain? Let’s create an automation that tells us what the weather is like in our locale and learn a little bit about the Slack automation platform while doing so.
What you will build
Your automation in Slack will contain:
- Built-in function: Collect info in a form
- Custom function: Weather function
- Built-in function: Send an ephemeral message with output of Step 2.
In this workshop, you’ll be using Workflow Builder, which is built right into Slack. Workflow Builder creates workflows which help you automate routine tasks and processes in Slack through the use of steps. Examples of these steps are Slack native functions like: creating a channel, sending a message or opening a form but they can also call upon third-party services. Let’s take a quick look at the final product that we’re going to build today.
While this workflow seems simple, it’s extremely powerful. We are using a weather service in this example but it shows off that you can connect any service that you want into Slack. Let’s build this together!
Step 1
Mise en place (Everything in its place)
Before we start, let’s make sure that we have all our tools in place in order to succeed. First, create a new sandbox by joining the Slack developer program, and create a testing channel.
1. Slack CLI Setup
Install the Slack CLI following these instructions.Make sure to choose the correct OS when installing the Slack CLI.
2. Test the Slack CLI installation
- In your terminal, run the command: slack version.
- If the installation was successful, you should see a version number displayed.
3. Connect Slack CLI with your Slack Workspace:
In the Codespace (or local) terminal, run: slack login. Follow the prompts to authenticate.
- Copy the command that looks like /slackauthticket THISISYOURTOKEN123456789 (including the /).
- Paste this command into any Slack channel. This will trigger a modal asking for permission.
- After accepting, copy the challenge code provided.
- Paste the challenge code back into your terminal.
You’re now authenticated! If you encounter any issues, refer to our documentation on authentication.
Step 2
Creating your Slack project
Before we create our Slack project, we should make sure that you’re in a location where you’re able to create folders.
Next, we can use a template by adding the --template
flag to the slack create command. This will use the provided Git repository to create your very own Slack project, which will contain all the files needed to run your function. This command is as follows:
slack create my-weather-app --template slack-samples/deno-function-template
Once this is done, head into the folder created by this command by using the change directory command:
cd my-weather-app
And opening your project up using VSCode with the following command:
code .
Step 3
Creating an account with OpenWeather
Last on our list of things to prepare is creating an account with openweathermap.org which is our service of choice here that we’ll be pulling weather information from. Follow the prompts on this page and once you’ve created an account, head over to the API keys tab and retrieve your API key, which we’ll be using later.
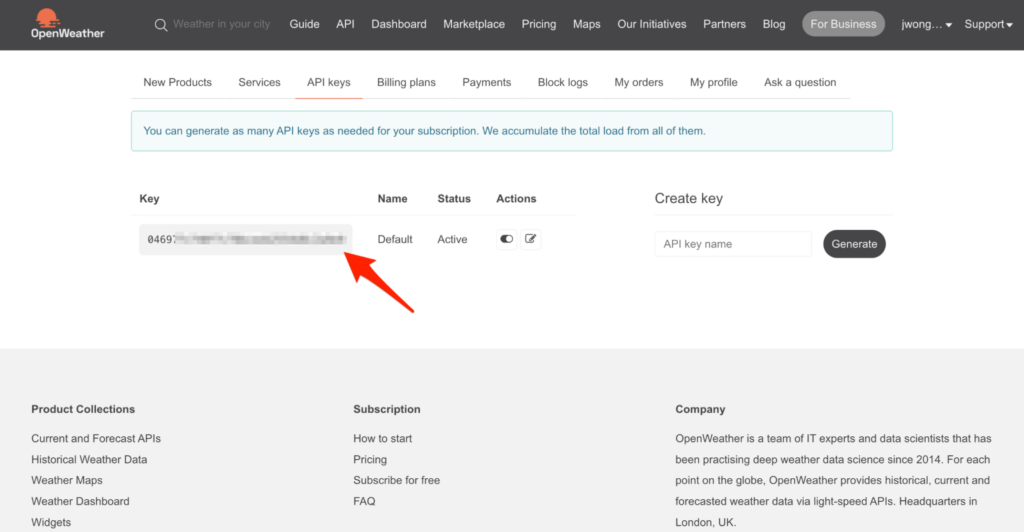
Step 4
Creating a workflow with Workflow Builder
Now that we have everything in place, let’s start building our workflow. During this process we’ll learn more about workflow steps and how to create a custom step for ourselves to integrate third party services.
First, let’s locate Workflow Builder within Slack. Click on your workspace name in the top left corner and scroll down to Tools and select Workflow Builder. Once you do this, you should see a new window pop up. This is where all of your workflows will live.
From here, click Create Workflow and choose From a link in Slack as your workflow start point. Normally, it would make sense that this workflow is run on a schedule but in order to test more easily, we’re going to use a link for the time being.
The next page you’ll see is your workflow’s main page. Take a moment here to update your workflow name to something other than Untitled Workflow.
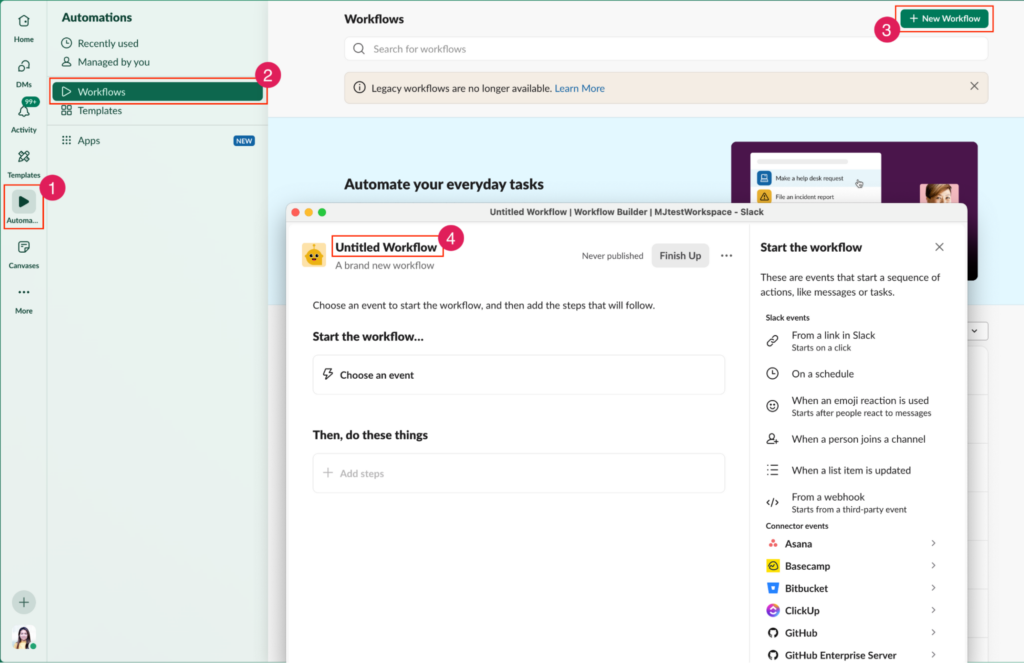
Step 5
Adding a form Workflow step
The first step that you’ll add to your workflow is a Form. You’ll find all of your steps in the right sidebar. Click Form and follow the prompts that show up on-screen. Within your form, you’ll want to add one question. Using a Single line of text, ask your users for the location that they want the weather for. After you add this question to your form, you’ll be able to access it as a variable in subsequent steps.
Step 6
Calling OpenWeather API
Your next step will call upon the powers of a service outside of Slack. In our particular case, we’ll be calling upon the OpenWeather API to get weather information from around the world. To help you along, the specific API method that should be called is the current weather data by city name . As for outputs, you’ll want to pull out the weather.description, which gives us a description of the weather in plain language.
Within your workflow, the foundation has already been laid for you to create your function. This function will show up within Workflow Builder as a step that you can add to any workflow, just like the Form step that we added earlier.
It is highly recommended to use what is already present to help you code your own function as there is a minimal amount of code needed to finish this workshop. Follow the TODOs listed here and make the corresponding changes within your sample_function.ts file.
import { DefineFunction, Schema, SlackFunction } from "deno-slack-sdk/mod.ts";
export const SampleFunctionDefinition = DefineFunction({
callback_id: "sample_function",
// TODO 1: Modify the `title` and `description` of your function so that you can refer to it later.
title: "Jason's weather function",
description:
"Jason's weather function that checks a location for the weather ",
source_file: "functions/sample_function.ts",
// TODO 2: These input parameters correspond to fields within your form, which are passed along to this step.
// Update this section to reflect the `location` parameter found within the form.
input_parameters: {
properties: {
your_input_parameter: {
type: Schema.types.string,
description: "your parameter description",
},
},
required: ["your_input_parameter"],
},
// TODO 3: Since your function will produce a single string output that can be used in your workflow,
// Update this section to reflect this with a field called `weather_result`.
output_parameters: {
properties: {
your_output_parameter: {
type: Schema.types.string,
description: "your parameter description",
},
},
required: ["your_output_parameter"],
},
});
export default SlackFunction(
SampleFunctionDefinition,
async ({
inputs,
}) => {
// TODO 4: Call this OpenWeather API method and retrieve the `weather.description` parameter from the JSON result.
// Set the parameter as `weather_result` and remember to add it as an output to this function
// by copying the code below.
const location = inputs.location;
const api_key = "";
const resp = await fetch(
`https://api.openweathermap.org/data/2.5/weather?q=${location}&appid=${api_key}`,
);
const weather_json = await resp.json();
// Use this to view the JSON object if needed!
// console.log(weather_json)
const weather_result = "";
return {
outputs: {
weather_result,
},
};
},
);
Step 7
Configuring your manifest.ts file
The manifest.ts file declares everything about your app, from which functions it hosts to which domains that your app will call. There are a few things that we’ll need to change here in order for you to be able to find your step within Workflow Builder.
- Change the name of your app to something like <your-name>-weather-function. e.g. jason-weather-function.
- Within the outgoingDomains array, add in the “api.openweathermap.org” domain.
Again, it is imperative that you do these two steps or else you will not be able to find your step nor call the OpenWeather API.
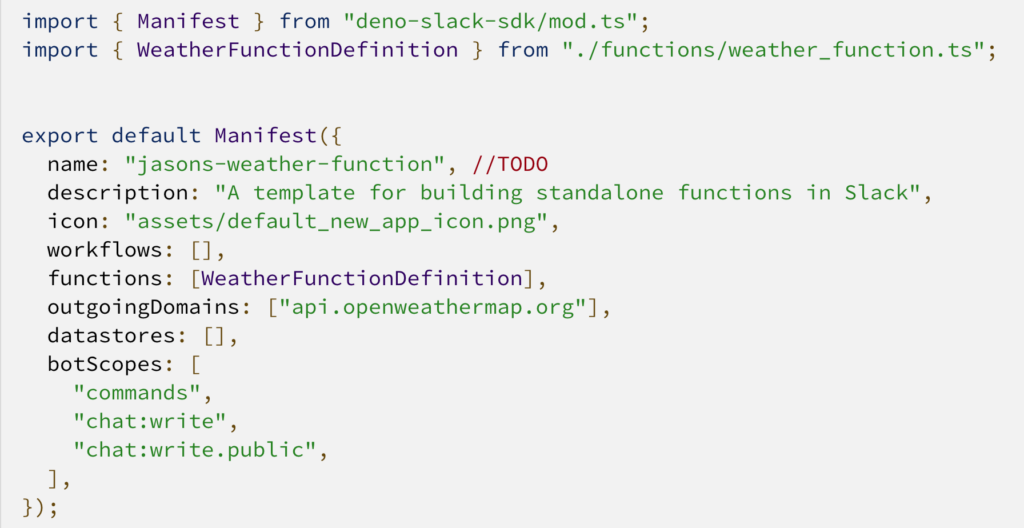
Step 8
Running your Slack app, and connecting it to the workflow
Now that you’ve finished coding your app, you might like to actually test it in the wild. In order to do so you can run your app locally by using the following command:
slack run
After following the prompts, a successful slack run execution will show a message like Connected, awaiting events in the Terminal window. This means that your app is up and ready to go — you will now see your step within Workflow Builder. Head back to your workflow page and add the step into your workflow by searching the step’s name in the right-hand sidebar. When adding the step into your workflow, make sure that you choose the answer to the location question from your form by clicking the {} beside the field box, do not enter the name of your city here or else it will not be variable.
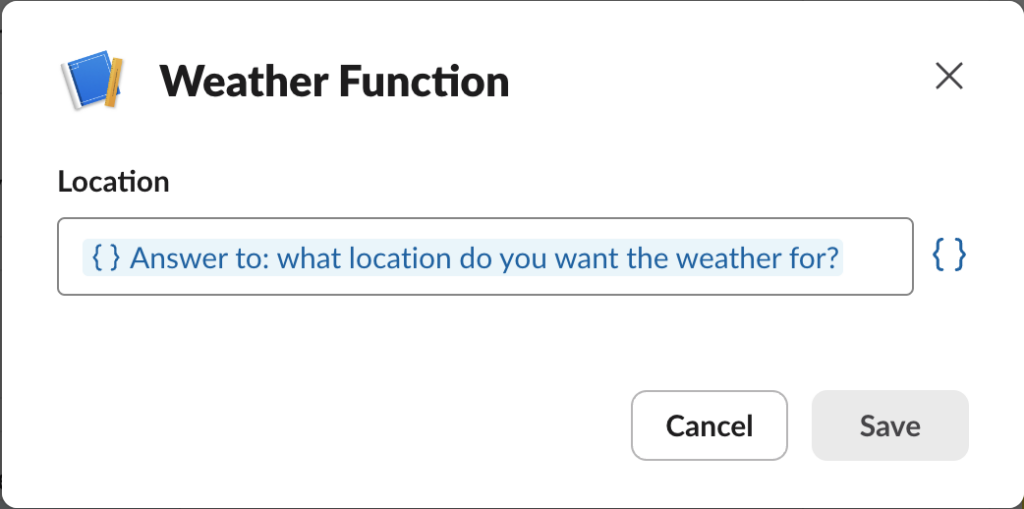
Step 9
Send a message with your weather result
Finally, you’ll want to send your user a message and notify them of the weather result so that they can make a decision to WFH or the office. On the right sidebar click on Messages > Send a “only to you” message. Write your message in the textbox and remember to include the variables {} in the message, as shown in the screenshot below. Make sure you’ve published your workflow by hitting the green Publish button in the top right-hand corner. As an example, your final workflow might look like this.
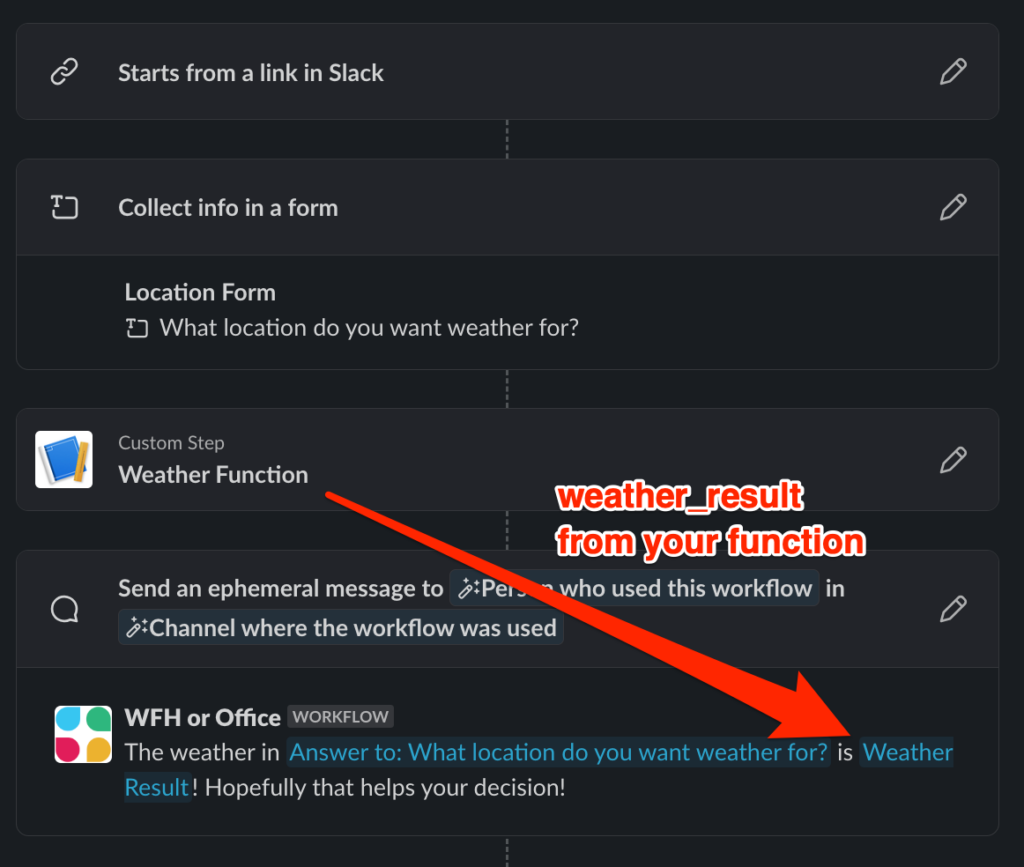
Once you’ve finished this, make sure that your workflow works to your liking by posting it into the testing channel and pressing the Start Workflow button.
Step 10
Extra credit (more learning!)
You may have noticed that we used our API key directly within our code and while this does indeed work correctly, it is best practice to hide these details from prying eyes by using environment variables. Rewrite your function to make use of the .env file for local variables (when running slack run) and also deploy these variables using the following command (for your deployed app, see step 11).
slack env add OPEN_WEATHER_MAP_API_KEY abcdefghijklmnopqrstuvwxyz123
Step 11
Deploy your weather app
We’ve used slack run
to run our app on our local machine, but as soon as you shut that environment down or turn off your computer, the function will stop running. Deploying your function to infrastructure managed by Slack ensures that the function is always available. Please note that there may be a cost associated with deploying functions to Slack, similar to using any serverless hosting environment (although it is completely for free when using the developer program sandboxes).
To deploy your function to production simply run: slack deploy
.
Choose the appropriate workspace for deployment. Remember, the local version (slack run
) and the production version (slack deploy
) are separate. Update the Workflow Builder to use the production function and reconnect input variables (step 8).