Build a daily random fact generator using BoltJS
Automate a 3rd-party API and learn useless facts
Difficulty
Intermediate
Time
30 minutes
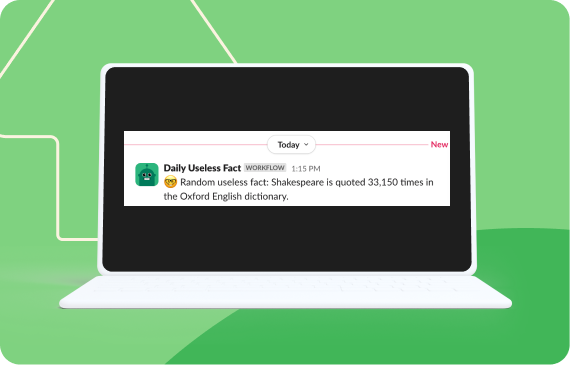
Requirements
- Visual Studio Code (or code editor of your choice)
- Node.js
- A testing workspace (get an Enterprise Grid sandbox for free by joining our developer program)
- Bolt for JavaScript
- Workflow Builder
Quick Jump
- Step 1: Create your new Slack app
- Step 2: Enable Socket Mode
- Step 3: Update your app manifest
- Step 4: Install your app
- Step 5: Let’s start coding!
- Step 6: Run your Bolt app
- Step 7: Create a new workflow in Slack using Workflow Builder
- Step 8: Schedule the workflow
- Step 9: Add your Bolt app’s function to the workflow
- Step 10: Add a step to send a message to a channel
- Step 11: Publish and test the workflow
- Step 12: (Optional) Make changes to the workflow or schedule
- Step 13: Deploying your Bolt app
Create a daily fact generator using a custom function and Workflow Builder
Do you have a monthly, weekly, or daily report you would like to automate? If the data you need is accessible with an API, with just a little programming you can open up a whole new world of unlimited possibilities!
In this workshop, we’ll guide you step by step to create a workflow that automates fetching data from a 3rd-party API and sending a formatted message to a channel with the results. As an example, we’ll use the random useless facts API to post a daily fact to a Slack channel. You’ll learn how to use the Bolt for JavaScript framework to add custom steps to your Slack workspace and incorporate into automations using Workflow Builder.
Let’s get started!
Step 1
Create your new Slack app
- Log in to your Slack workspace or join the Developer Program to get a free enterprise sandbox.
- Open Your Apps dashboard.
- Click Create New App > From scratch.
- Add a name for your app, such as “Useless Fact App” (you can update it later).
- Select the workspace where you want to install your app (e.g., the sandbox you created).
- Click Create App.
Step 2
Enable Socket Mode
Your app will use Socket Mode to connect to Slack using a dynamic, two-way communication. This simplifies the setup for developing and testing new applications.
- Click Socket Mode on the sidebar.
- Toggle Enable Socket Mode on.
- To enable Socket Mode, you must generate an app-level token. Type “useless-fact-app-token” for the Token Name.
- Click Generate.
- The new app level token is displayed. You will need this token, but you can copy and paste it in a later step. Click Done.
Step 3
Update your app manifest
All apps built for Slack have an app manifest. This is the configuration for the app, such as the name, settings, and required permissions. The app manifest also describes any functions (custom code) your app will make available to your Slack workspace. It’s important to note the following app manifest includes a function named useless_fact_step and declares the function returns one value named “Fact” as its output.
- Click App Manifest on the sidebar.
- Copy the following app manifest and update your default app manifest. Update the name “Useless Fact App” if you used a different name.
{ “display_information”: {“name”: “Useless Fact App”
},
“features”: {
“app_home”: {
“home_tab_enabled”: true,
“messages_tab_enabled”: true,
“messages_tab_read_only_enabled”: true
},
“bot_user”: {
“display_name”: “Useless Fact App”,
“always_online”: false
}
},
“oauth_config”: {
“scopes”: {
“bot”: [
“chat:write”,
“app_mentions:read”,
“workflow.steps:execute”
]
}
},
“settings”: {
“event_subscriptions”: {
“user_events”: [
“message.app_home”
],
“bot_events”: [
“app_mention”,
“workflow_step_execute”,
“function_executed”
]
},
“interactivity”: {
“is_enabled”: true
},
“org_deploy_enabled”: true,
“socket_mode_enabled”: true,
“token_rotation_enabled”: false,
“hermes_app_type”: “remote”,
“function_runtime”: “remote”
},
“functions”: {
“useless_fact_step”: {
“title”: “Useless Fact Custom Step”,
“description”: “Runs useless fact step”,
“input_parameters”: {},
“output_parameters”: {
“message”: {
“type”: “string”,
“title”: “Fact”,
“description”: “A random useless fact”,
“is_required”: true,
“name”: “message”
}
}
}
}
}
- Save your changes.
Step 4
Install your app
Now, install your app to your Slack workspace (sandbox).
- Navigate to Install App in the sidebar.
- Click Install to <Name of your workspace>.
- Approve the installation and scopes, and it will generate the Bot User token. You will need this token for the app to work, but you can copy it in a later step.
Step 5
Let’s start coding!
Now that you’ve completed the app setup, it’s time to write some code!
- Open your terminal or command prompt.
- First, check to see that you have Node.js installed and it is a recent long-term support (LTS) version by typing the following command.
node -v
If you get an error or if it’s an older version than what’s available for download on the Node.js website, take a moment to install the latest version. - Create a new directory for the project named useless-fact-app and change to the new directory.
mkdir useless-fact-app
cd useless-fact-app
(If you’re on Windows, the command is: md useless-fact-app) - Initialize a new Node.js project using the following command and answer each question with defaults.
npm init - Our project is going to use two packages: Slack’s Bolt for JavaScript framework and axios, a popular HTTP client for making API calls. Install these dependencies with the following command.
npm install @slack/bolt axios - Open the project in Visual Studio Code or your favorite code editor.
- Create a new file in the project folder named .env, open the file and paste the following.
SLACK_SIGNING_SECRET={your-app-signing-secret}
SLACK_BOT_TOKEN={your-bot-token-xoxb-1234}
SLACK_APP_TOKEN={your-app-token-xapp-1234}
PORT=3000 - Return to the Slack app dashboard and find the values needed for the .env file. Click on Basic Information, click on Show by the Signing Secret, and copy this value. Replace {your-app-signing-secret} in the .env file with your Signing Secret.
- Scroll down and click on the name of your app-level token, then click Copy. Replace {your-app-token-xapp-1234} in the .env file with your app-level token.
- Click OAuth & Permissions. Find your Bot User OAuth Token, and click Copy. Replace {your-bot-token-xoxb-1234} in the .env file with your Bot User token.
- Edit the package.json file, update the following settings, and save the file.
Change “main” to:
“main”: “app.js”,
Change the “scripts” to:
“scripts”: {
“dev”: “node –env-file=.env –watch app.js”
},
Change “type” to “module” (or add this line if not already present):
“type”: “module”, - Create a new file named app.js and add the following code.
import bolt from “@slack/bolt”;
import axios from “axios”;
const { App } = bolt;
// Initialize the Bolt app
const app = new App( {
token: process.env.SLACK_BOT_TOKEN,
signingSecret: process.env.SLACK_SIGNING_SECRET,
appToken: process.env.SLACK_APP_TOKEN,
socketMode: true
} );
// Make an API call to retrieve a random fact
async function getUselessFact() {
const res = await axios.get( “https://uselessfacts.jsph.pl/api/v2/facts/random” );
return ( res.status === 200 ) ? res.data : null;
}
// Define the function for Workflow Builder
app.function( “useless_fact_step”, async ( { complete, fail, logger } ) => {
try {
app.logger.info( “Running useless fact step…” );
// Get the fact using the API
const fact = await getUselessFact();
if ( fact && fact.text ) {
// Use complete() to send results to Slack
await complete( {
outputs: {
message: fact.text
}
} );
} else {
// Use fail() to send an error to Slack
await fail( { error: “There was an error retrieving a random useless fact :cry:” } );
}
} catch ( error ) {
// Log the error and send an error message back to Slack
logger.error( error );
await fail( { error: `There was an error 😥 : ${ error }` } );
}
} );
// Start the Bolt App!
async function main() {
await app.start( process.env.PORT || 3000 );
app.logger.info( “⚡️ Bolt app is running!” );
}
main();
The code in app.js initializes the Bolt app using your tokens and signing secret and enables socket mode. Next it defines the getUselessFact() function that retrieves a random fact using axios. Then it uses the Bolt framework to create a Slack function named useless_fact_step. This is the same function we registered in the app manifest in step 3, and it’s important when creating functions that the code and app manifest match on name, inputs, and outputs. Finally, a function named main() is used to start the Bolt app.
Step 6
Run your Bolt app
From your terminal, enter the following command.
npm run dev
You should see the following printed to the console.
> bolt-useless-facts@0.1.0 dev > node --env-file=.env --watch app.js [INFO] bolt-app ⚡️ Bolt app is running!
Your Bolt app is running, and you can now use it in your Slack workspace!
Step 7
Create a new workflow in Slack using Workflow Builder
To create a new workflow, you will need to open Workflow Builder in Slack. You can open Workflow Builder using one of the following methods.
Option 1: Use the message box
In any channel, write: /workflow and select Create a new workflow
Option 2: Use the sidebar
- Navigate to the left sidebar and click More > Automations > Workflows
- Click +New Workflow > Build Workflow
Step 8
Schedule the workflow
Every workflow starts with a trigger. For this workflow, it may make sense to have it scheduled for each work day at a specific time.
- Under Start the workflow… click Choose an event
- From the list of events, click On a schedule
- Configure the schedule for your app to run. Under Starts on, choose today’s date. Change the time to 15 or 30 minutes from now (you can update the scheduled time and frequency after you verify it is working). Under Frequency, choose Every weekday (Monday to Friday). Click Continue.
Step 9
Add your Bolt app’s function to the workflow
After the workflow is triggered, the next step is to call the function in the Bolt app. This is done using a custom step.
- Click + Add steps.
- Click Search steps…
- In the search box, start typing the name of your Bolt app.
- Click Useless Fact Custom Step.
- Click Save.
Step 10
Add a step to send a message to a channel
After calling the Bolt app and retrieving a random fact, the next step is to send a message to a channel.
- Click + Add Step.
- Click Messages and then click Send a message to a channel.
- Click Select a channel and choose a channel to send a message.
- Under Add a message, type something like “🤓Random useless fact:”
- Click {} Insert a variable and then select Fact under 1. Useless Fact Custom Step.
- Click Save.
Step 11
Publish and test the workflow
Almost there! The final task is to publish the workflow and wait for the next scheduled run to see it in action!
- Click Finish Up.
- Enter a Name, such as “Daily Useless Fact”
- Update the Description.
- Click Publish.
- Wait for the scheduled time and see your new custom Bolt app in action!
Step 12
(Optional) Make changes to the workflow or schedule
If needed, you can always make changes to your workflow, such as the schedule, channel, or message.
- Click More -> Automations -> Workflows.
- Find your workflow and click the three vertical dots on the right side.
- Click Edit workflow.
Step 13
Deploying your Bolt app
Congratulations! You’ve learned how to create a Bolt app using the Bolt for JavaScript framework and a daily scheduled workflow using Workflow Builder!
The Bolt app will continue to work as long as you have it running on your computer. This is fine for development and testing purposes, but a better long-term solution is deploy the application to a production environment. You can follow the guides available for Deploying to AWS Lambda or Deploying to Heroku for steps on deploying to those environments, or deploy to any platform that supports JavaScript serverless functions or Node.js applications.